Overview of Custom Paint and Canvas
Custom Paint is a widget in Flutter that provides a canvas on which to draw during the paint phase. It allows developers to create custom graphics, shapes, and other visual elements by directly painting onto the screen.
Key Features:
- Custom Painter: CustomPaint uses a CustomPainter to paint on the canvas. The CustomPainter class must be extended to define the painting logic.
- Size: The size of the CustomPaint widget can be controlled and is passed to the CustomPainter for drawing.
- Child Widget: CustomPaint can have a child widget, which is painted after the custom drawing is done.
Canvas
Canvas is the core class in Flutter for drawing 2D graphics. It provides methods to draw shapes, text, images, and paths.
Key Methods:
- drawLine: Draws a line between two points.
- drawRect: Draws a rectangle.
- drawCircle: Draws a circle.
- drawOval: Draws an oval.
- drawPath: Draws a path defined by a series of points.
- drawImage: Draws an image.
- drawText: Draws text on the canvas.
- drawArc: Draws an arc.
How to Use Custom Paint and Canvas
Create a Custom Painter Class:
- Extend the Custom Painter class and override the paint and should Repaint methods.
- The paint method contains the painting logic using the Canvas and Size objects.
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('Custom Paint Example')),
body: Center(
child: CustomPaint(
size: Size(200, 200),
painter: CirclePainter(),
),
),
),
);
}
}
class CirclePainter extends CustomPainter
@override
void paint(Canvas canvas, Size size) {
final paint = Paint()
..color = Colors.blue
..strokeWidth = 4
..style = PaintingStyle.stroke;
final center = Offset(size.width / 2,
size.height / 2);
final radius = size.width / 2;
canvas.drawCircle(center, radius, paint);
}
@override
bool shouldRepaint(CustomPainter oldDelegate) {
return false;
}
}
The should Repaint method in Custom Painter is an important part of the custom painting process in Flutter. It determines whether the Custom Painter should repaint its contents when the widget’s configuration changes.
Purpose
The should Repaint method is used to optimize the painting process by avoiding unnecessary repaints. If the method returns true, Flutter will call the paint method to redraw the custom graphics. If it returns false, the existing painting is reused, which can improve performance.
Parameters
- oldDelegate: The previous CustomPainter instance that was used to paint the widget. You can compare this with the current painter to determine if any properties that affect the painting have changed.
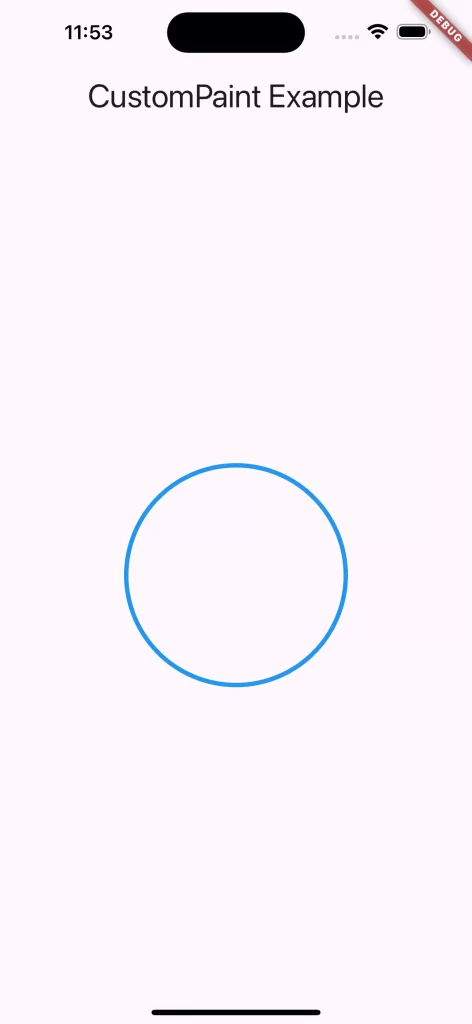
Drawing Shapes and Paths
- How to draw various shapes like rectangles, ovals, and paths.
class PathPainter extends CustomPainter {
@override
void paint(Canvas canvas, Size size) {
final paint = Paint()
..color = Colors.red
..strokeWidth = 4
..style = PaintingStyle.stroke;
final path = Path()
..moveTo(size.width / 2, size.height / 2)
..lineTo(size.width, size.height)
..lineTo(size.width, 0)
..close();
canvas.drawPath(path, paint);
}
@override
bool shouldRepaint(CustomPainter oldDelegate) {
return false;
}
}
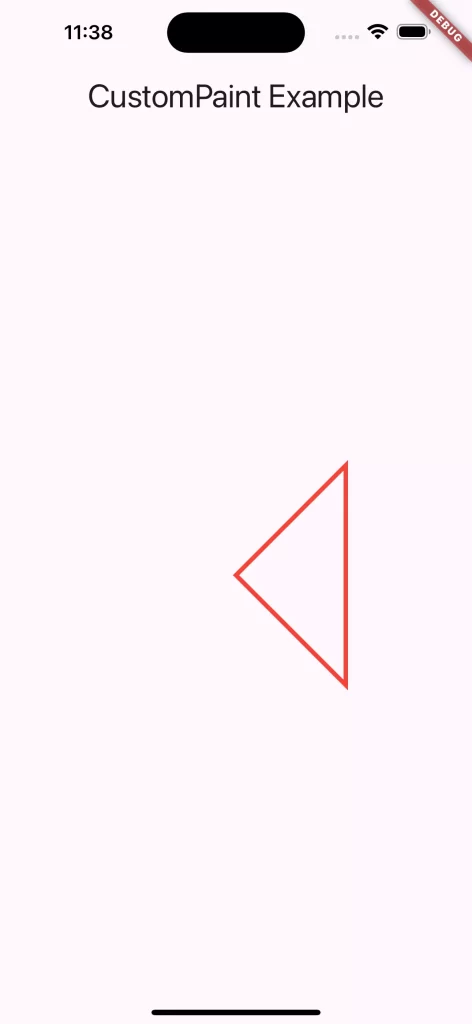
Using Gradients and Shadows
- Adding gradients and shadows to custom drawings.
class GradientPainter extends CustomPainter {
@override
void paint(Canvas canvas, Size size) {
final paint = Paint()
..shader = LinearGradient(
colors: [Colors.blue, Colors.purple],
).createShader(Rect.fromLTWH(0, 0, size.width, size.height));
final rect = Rect.fromLTWH(0, 0, size.width, size.height);
canvas.drawRect(rect, paint);
}
@override
bool shouldRepaint(CustomPainter oldDelegate) {
return false;
}
}
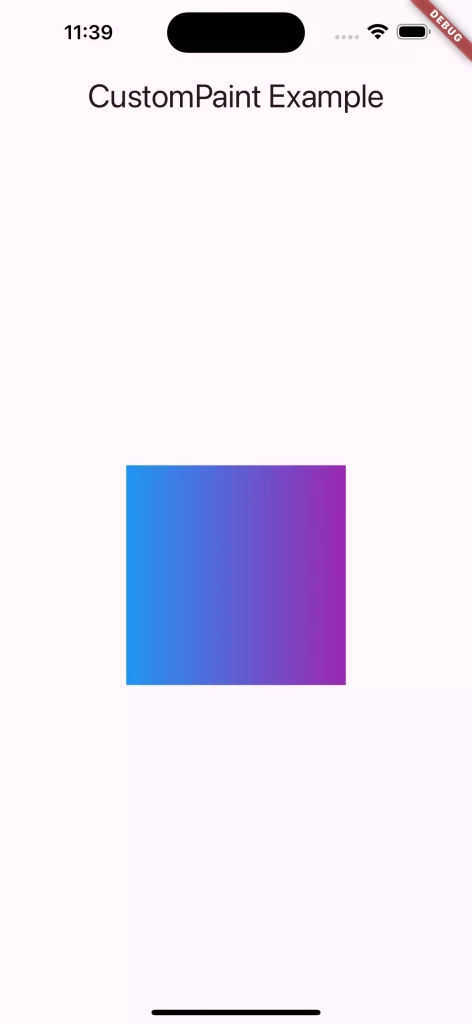
- Links to official Flutter documentation, tutorials, and community resources on custom painting.
Official Documentation:
A. Flutter Custom Paint Class – Official documentation for the Custom Paint class in Flutter.
B. Flutter Custom Painter Class – Official documentation for the Custom Painter class in Flutter rendering.
Tutorials and Guides:
- Flutter Custom Painting – Guide on custom painting in Flutter from the official Flutter website.
- CustomPaint in Flutter: Drawing Custom Shapes – Medium article explaining the basics of CustomPaint and drawing custom shapes.
- Drawing Custom Shapes in Flutter with CustomPainter – Tutorial on how to draw custom shapes using CustomPainter.
- Community Resources:
- Flutter Community – Official Flutter community page with links to forums, social media, and events.
- Flutter GitHub Repository – Explore Flutter’s GitHub repository for issues, discussions, and contributions related to custom painting and other Flutter topics.
These resources should provide you with a solid foundation to learn and master custom painting techniques in Flutter.
Conclusion
- Summary of Key Points
- CustomPaint and CustomPainter provide powerful tools for creating custom graphics in Flutter apps.
- Examples demonstrated basic shapes, complex paths, animations, and interactive drawings.